Use case
This use case describes the integration of Salesforce with the Goods and Services Tax portal. This integration allows Salesforce users to authenticate and easily access information about GST return filings and ledger-related data from the Salesforce platform itself.
Solution design
The GST portal does not directly provide API services; there are authorised intermediaries and intermediaries called GSP (GST Suvidha Providers) to provide access to the GST portal.
To integrate Salesforce with the GST portal, follow these high-level steps
- Find an appropriate GST Suvidha Provider (GSP) and open an account with them.
- Look at the GSP’s API methods to determine which are relevant for obtaining the GST return filings and ledger information.
- Establish a data model in Salesforce to store the retrieved data. Create an Apex class in Salesforce to handle API calls, authentication, and data storage. Execute the integration by invoking the Apex class into a screen flow or custom component.
- Test the integration thoroughly to ensure proper functionality and data accuracy.
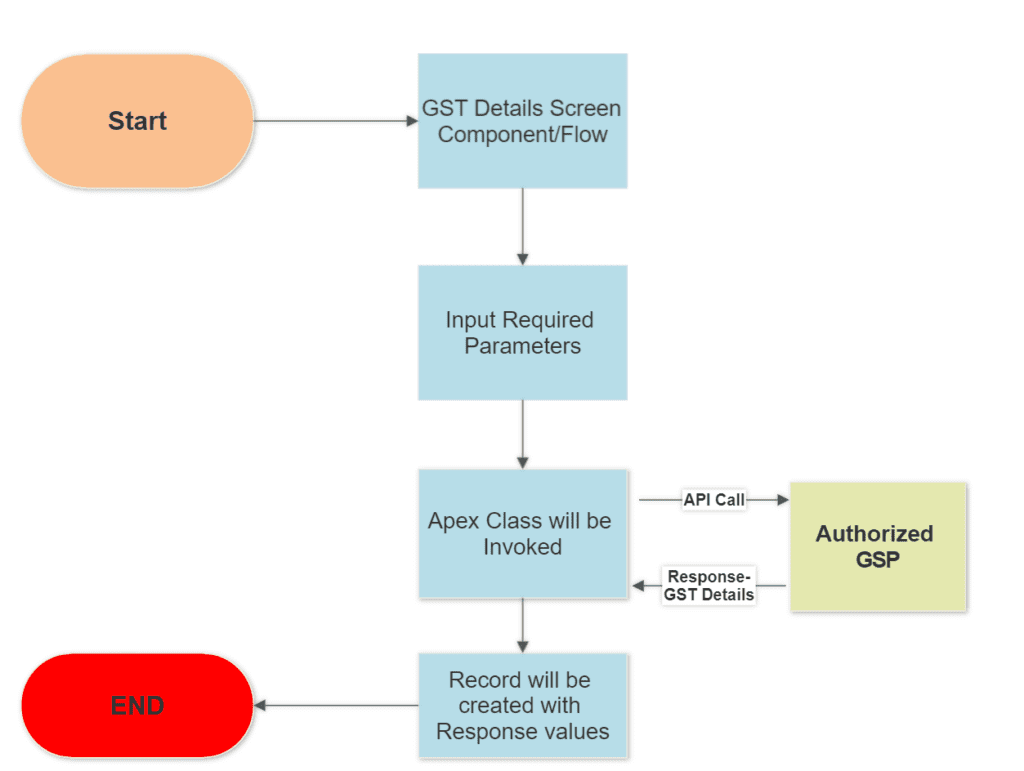
Implementation specifics
Identify the GSP
Conduct research and select a GSP like MasterGST. Then, sign up with them, create an account, and find out about their pricing and services.
Determine the API methods: Refer to the GSP’s documentation (in this case, MasterGST) to find out which API methods are available for accessing ledger-related data and GST return filings. Understand the functionality and parameters of each API method to retrieve the required data effectively.
Determine the data model in Salesforce
- In Salesforce, create custom objects and custom fields to store the retrieved GST data.
- Create the data model using the specific data elements provided by the GSP’s API.
- Ensure that the data is properly organised and stored within Salesforce.
Create an Apex Class
- In Salesforce, create an Apex Class to interact with GSP’s API.
- Implement the procedures within the Apex Class to make API calls, retrieve data, and handle responses.
- Save the GST data that was retrieved into the appropriate Salesforce objects and fields.
Implement integration
In Salesforce, create a user interface with Screenflow or Lightning Web Component (LWC) to collect the necessary input from the user. This input may include authentication credentials, API parameters, or other information required to access the GSP’s API in order to initiate the API call to the GSP’s system and invoke the previously created Apex class while passing the collected parameters.
Test and validate
Thoroughly test the integration to ensure that everything works as expected. Various scenarios should be tested, such as successful API requests, error handling, and data mapping.
Code snippet (high-level)
Here is a high-level code snippet for retrieving taxpayer details using the masterGST rest API. The GSTIN will be passed from the screen flow (user interface) input fields, as shown in the code below.
Http http = new Http();
HttpRequest request = new HttpRequest();
request.setEndpoint(MASTER_GST_API+'/public/search?email='+EMAIL_ID+'&gstin='+GSTIN);
request.setMethod('GET');
request.setHeader('client_id', ‘YOUR CLIENT_ID’);
request.setHeader('client_secret', ‘YOUR CLIENT_SECRET’);
HttpResponse response = http.send(request);
if(response.getStatusCode() == 200) {
System.debug('Response: ' + response.getBody());
string res = response.getBody();
Map<String, Object> responseval = (Map<String, Object>) JSON.deserializeUntyped(response.getBody());
Map<String, Object> responseob = (Map<String, Object>) responseval.get('data');
if (responseob != null) {
custom_obj newGSTdetails = new custom_obj();
String Type = (String)responseob.get('dty');
String Code = (String)responseob.get('stjCd');
String Building = (String)responseob.get('bnm');
String gstinNumber = (String)responseob.get('gstin');
newGSTdetails.Taxpayer_Type__c = Type;
newGSTdetails.State_Jurisdiction_code__c = Code;
newGSTdetails.Building_name__c = Building;
newGSTdetails.GST_Identification_Number__c = gstinNumber;
insert newGSTdetails;
} else {
System.debug('GSTIN data not found in response: ' + response.getBody());
}
}
The provided code snippet demonstrates an HTTP GET request from Salesforce to the MasterGST API. The request includes parameters like email and GSTIN to search for specific data. The code checks the response status code; if it is 200 (indicating a successful response), it retrieves and processes the response body. In JSON format, the response body is deserialised into a map structure. From the response, useful information is taken out and put into the right fields in a Salesforce object. This includes the type of taxpayer, the state jurisdiction code, the name of the building, and the GST identification number. Finally, the object is inserted into Salesforce. If the response does not contain the expected data, an appropriate message is displayed in the debug log.
Final thoughts
Integrating Salesforce with the GST portal necessitates the use of an authorised GST Suvidha Provider (GSP) as an intermediary. The GSP provides access to the GST portal through its API services. By following the outlined solution design, including steps such as identifying the GSP, setting up an account, defining a data model in Salesforce, creating an Apex class to handle API calls, and implementing the integration in Salesforce, you can establish a connection between Salesforce and the GST portal.